componentDidUpdate() in ReacJS Class-Based and Functional components
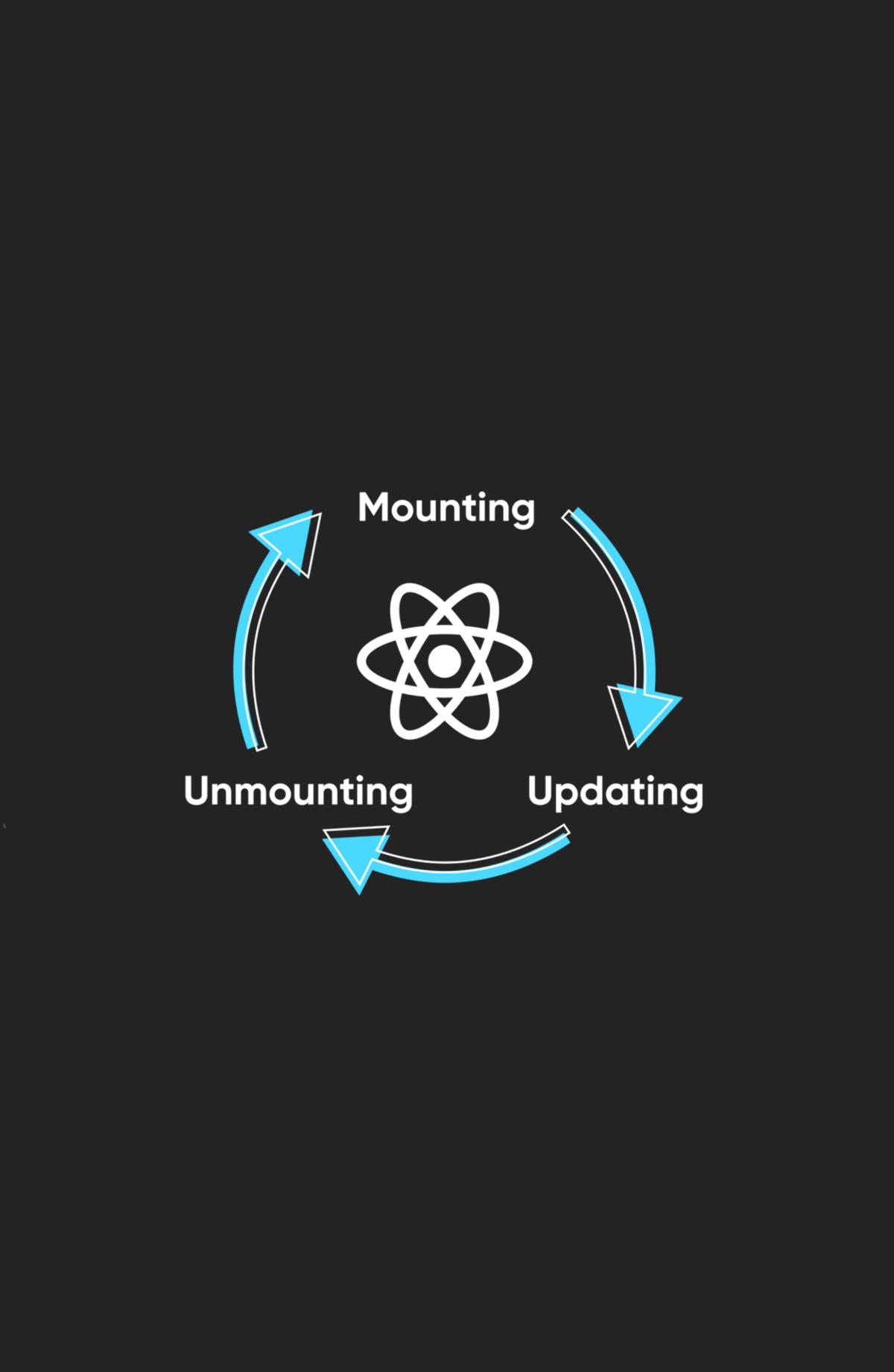
For Class-Based components
componentDidUpdate()
is a method that you can use when you need to perform some action or side effect after the component has updated due to changes in props or state. Here's an example demonstrating how you might use componentDidUpdate() in a class-based component:
In this example:
- We have a component MyComponent with a state variable count.
- When the button is clicked, the count state is incremented.
- In componentDidUpdate(), we compare the current count with the previous count. If they are different, we log a message indicating that the count has changed.
For Functional components
We can use hooks, in this case we're gonna use useEffect()
. If we pass an attribute in the array type parameter to the function, it will observe and react every time the attribute or attributes change
We have rewritten the same example using functional components and hooks:
In this functional component example:
- We use the useState() hook to manage the count state.
- We use the useEffect() hook with a dependency array containing count. This ensures that the effect runs after every render only if the count state has changed.
- Inside the effect, we log a message indicating that the count has changed, along with the current count value.
This functional component with hooks achieves the same behavior as the class component with componentDidUpdate()
, but with a simpler and more concise syntax.