componentDidMount() in ReacJS Class-Based and Functional components
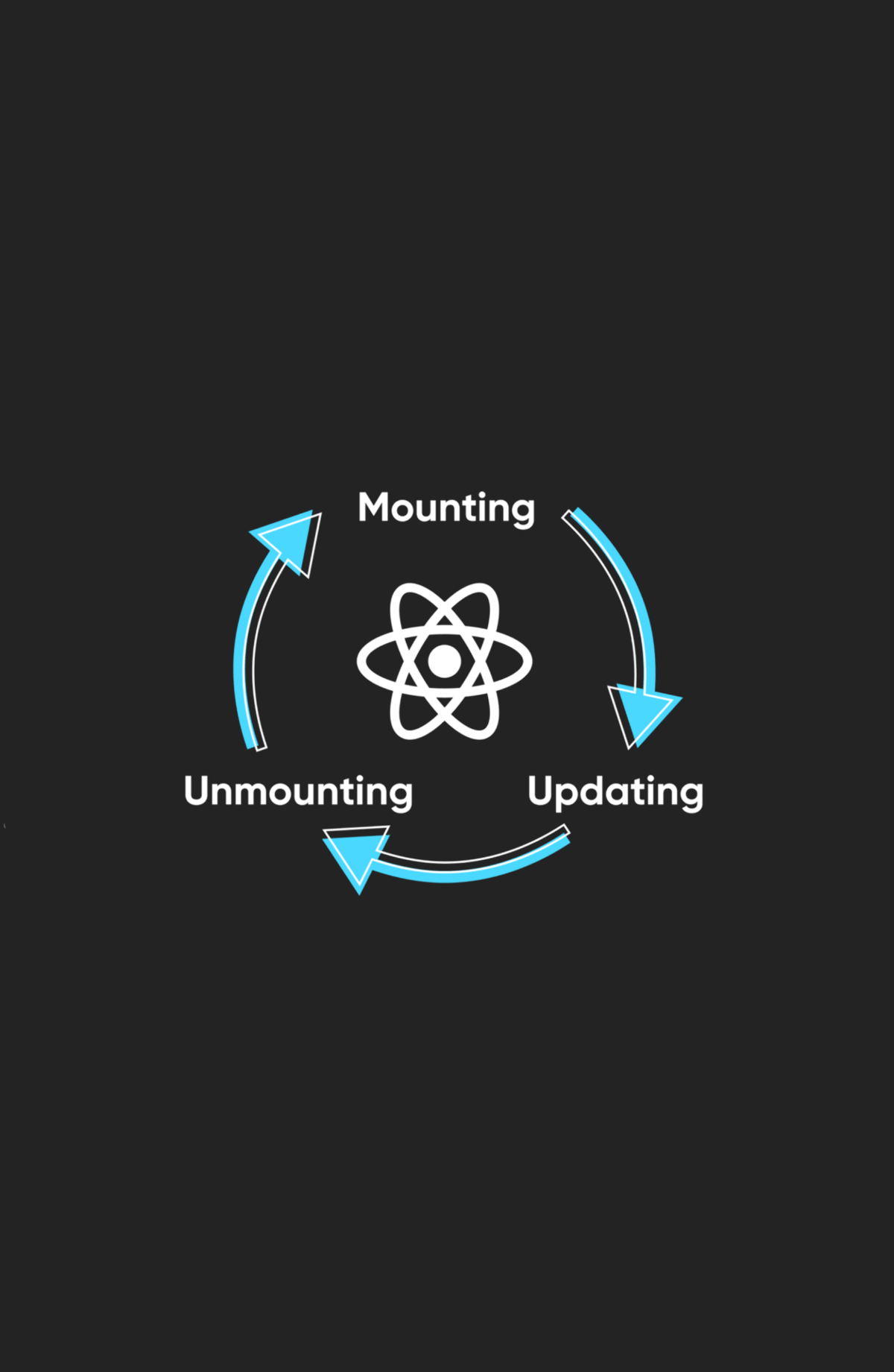
For Class-Based components
componentDidMount()
is a lifecycle method in React class components that gets called after the component has been mounted (i.e., inserted into the DOM). It's commonly used for performing tasks that require DOM manipulation or data fetching after the component has been rendered for the first time. Here's an example of how componentDidMount()
can be used:
In this example:
-
In the constructor, we initialize the component state with data set to null and isLoading set to true.
-
In componentDidMount(), we simulate an asynchronous operation (such as fetching data from an API) using setTimeout() for demonstration purposes. After 2 seconds, the data state is updated with a sample message, and isLoading is set to false.
-
In the render() method, we conditionally render a loading message if isLoading is true, and render the fetched data once it's available.
This is a basic example, but componentDidMount() is commonly used for tasks like fetching data from APIs, subscribing to events, initializing third-party libraries, and setting up timers. It's important to remember that any side effects performed in componentDidMount() should be cleaned up in componentWillUnmount() to avoid memory leaks or stale data.
For Functional components
componentDidMount() Equivalent
In functional components, useEffect()
with an empty dependency array ([]) serves as a replacement for componentDidMount()
. This effect runs once after the initial render, simulating the behavior of mounting.
In this functional component:
- We're using the useState() hook to manage component state. We initialize data with null and isLoading with true.
- We use the useEffect() hook with an empty dependency array ([]) to replicate the behavior of componentDidMount(). This effect runs only once after the initial render.
- Inside the useEffect() callback, we define a function fetchData() to simulate fetching data from an API using setTimeout(). After 2 seconds, we update the state with a sample message and set isLoading to false.
- We return a cleanup function from useEffect(). Although not needed in this example, it's good practice for cleaning up resources like event listeners or subscriptions to avoid memory leaks.
Overall, the functional component with hooks achieves the same functionality as the class component with componentDidMount(), but with more concise syntax and better readability.