ReactJS Path
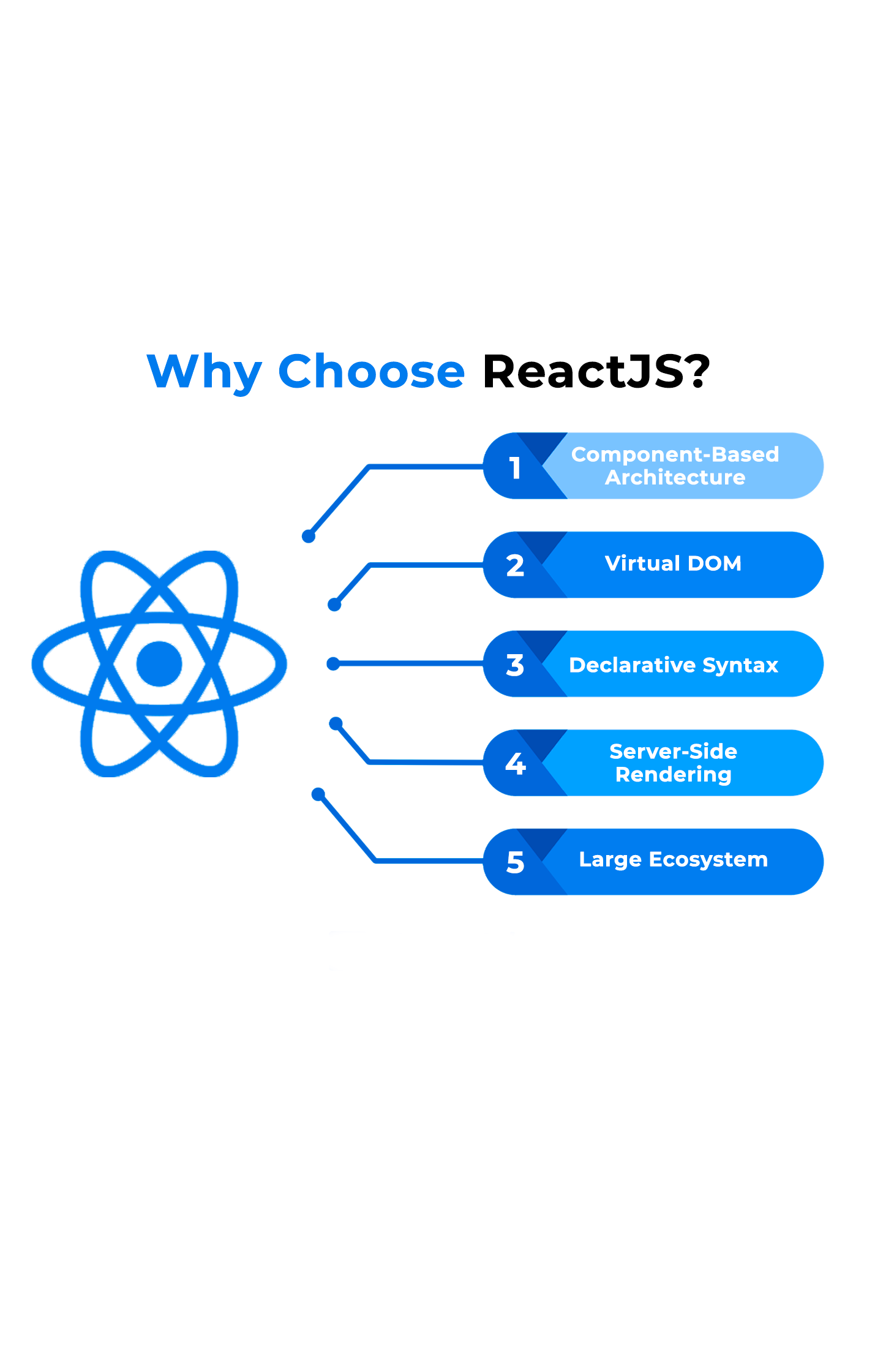
- Basic Concepts:
- Virtual DOM
- JSX (JavaScript XML)
- Components
- Props and state
- Lifecycle methods
- Componentization:
- Functional components vs. Class components
- Component composition
- Higher-order components (HOCs)
- Render props
- State Management:
- useState and useEffect hooks
- Context API
- Redux or MobX for more complex state management
- Routing:
- React Router for client-side routing
- Nested routes
- Route parameters and query strings
- Forms:
- Controlled vs. uncontrolled components
- Form handling and validation
- HTTP Requests:
- Fetch API or Axios for making HTTP requests
- Handling asynchronous data fetching
- Styling:
- CSS modules
- Styled-components or Emotion for CSS-in-JS
- Testing:
- Unit testing with Jest and Enzyme or React Testing Library
- Integration testing
- Snapshot testing
- Performance Optimization:
- Code splitting
- Memoization
- Virtualized lists
- Deployment:
- Create React App (CRA) for quick setups
- Deployment strategies for React applications (e.g., AWS, Heroku, Netlify)
- Advanced Topics:
- Server-side rendering (SSR) with Next.js or Gatsby
- React Suspense and React.lazy for code splitting
- Error boundaries
Make sure to review and practice these topics to feel confident in your React.js interviews!