Lifecycle in ReactJS
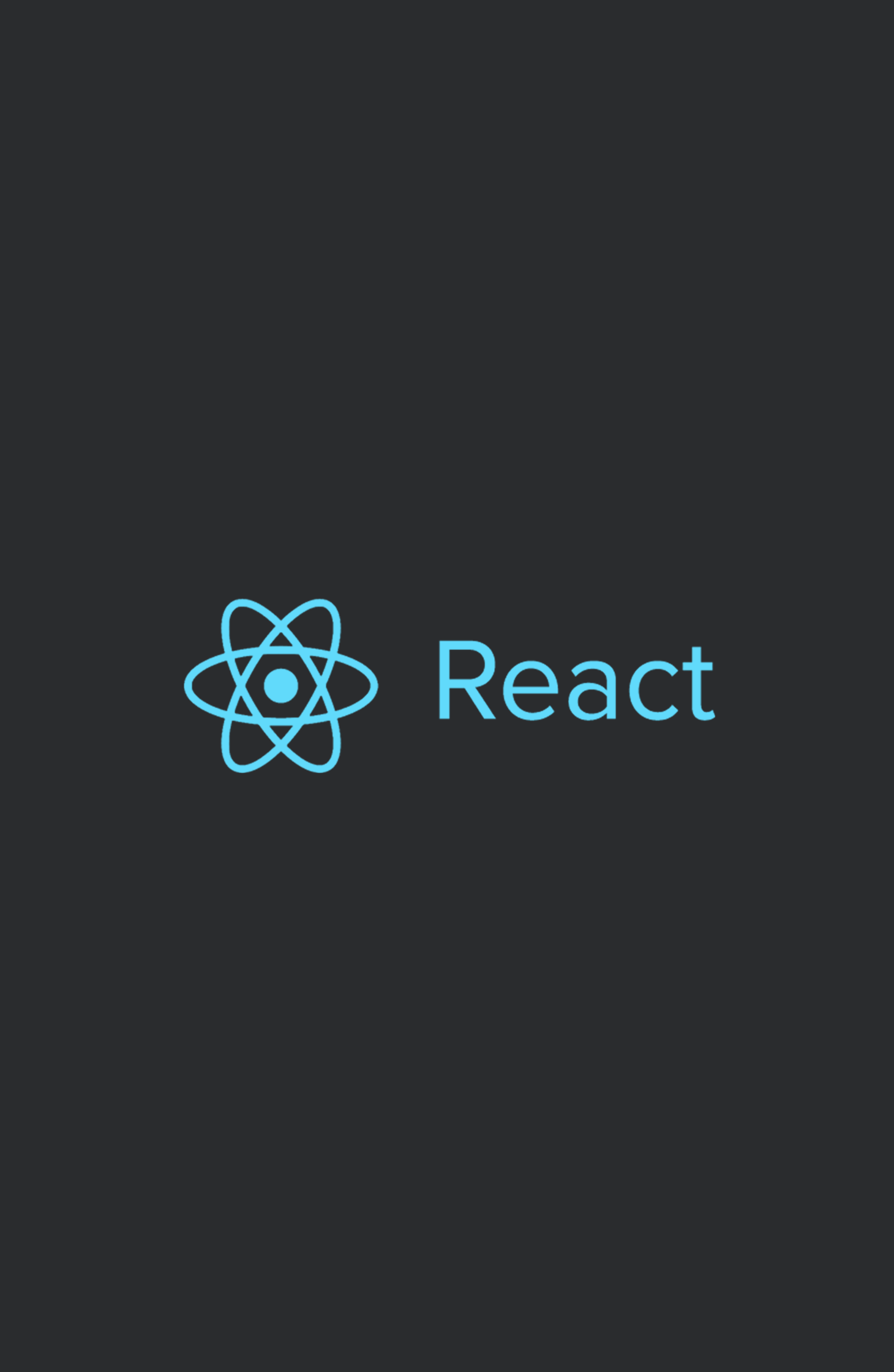
Hello there we're gonna present the lifecycle in ReactJS. Overall, lifecycle methods are special methods that get called at various points in the lifecycle of a component, from initialization to destruction.
With the introduction of React Hooks, managing component lifecycle has become more straightforward. Here's a comparison between traditional class-based lifecycle methods and equivalent functionality achieved with React Hooks:
Class-Based Components (Lifecycle Methods)
1.componentDidMount()
:
- Invoked immediately after a component is mounted (inserted into the tree).
- Commonly used for initializing state or making API calls.
More information and concepts related to this, check this post:
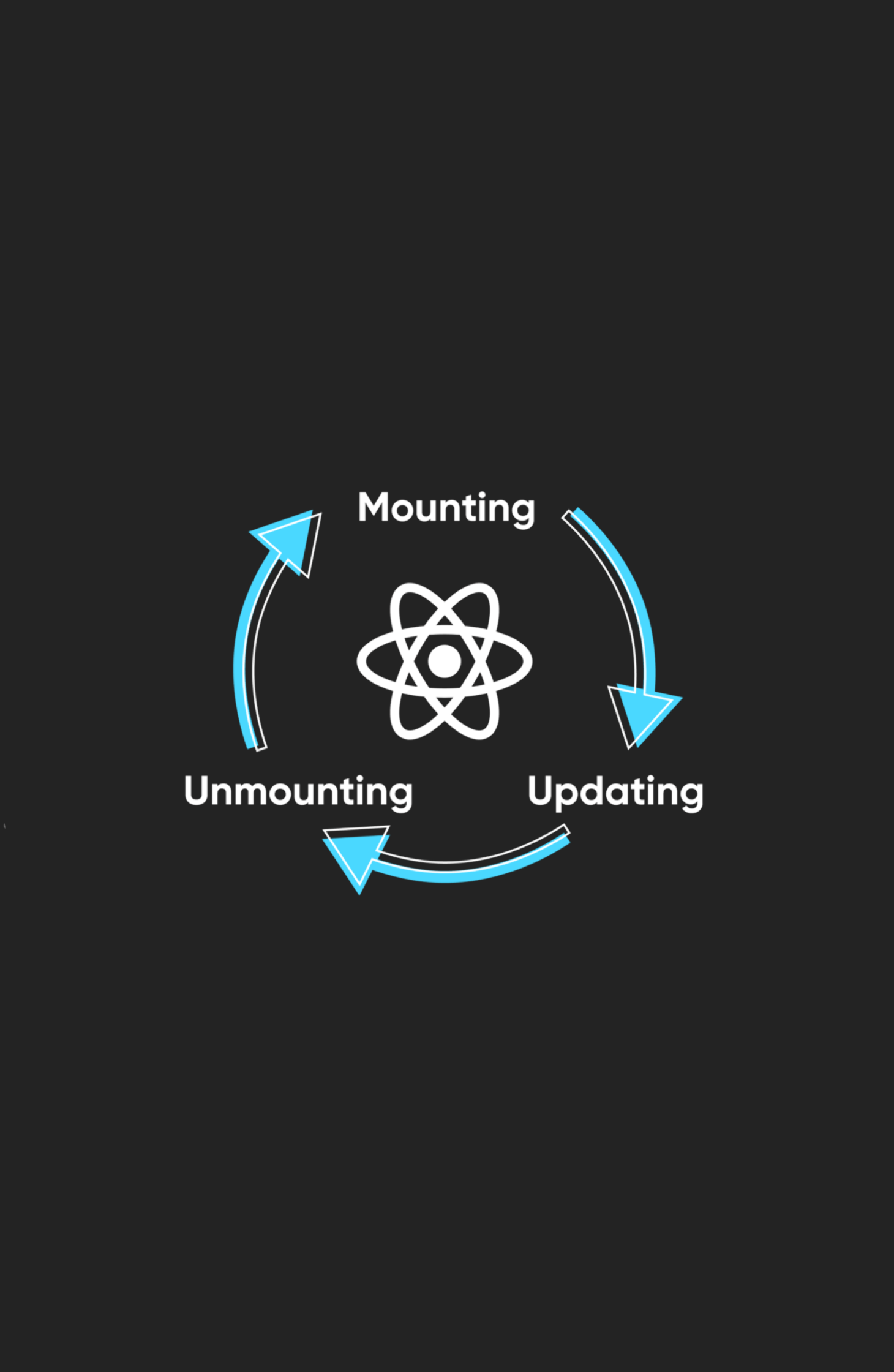
2.componentDidUpdate(prevProps, prevState)
:
- Invoked immediately after updating occurs.
- Commonly used to perform side effects in response to prop or state changes.
3.componentWillUnmount()
:
- Invoked immediately before a component is unmounted and destroyed.
- Commonly used for cleanup such as canceling timers or disposing of resources.
Functional Components with React Hooks (Equivalents)
useEffect()
could be equivalent to componentDidMount and componentDidUpdate
1. useEffect()
(componentDidMount):
- Runs after every render.
- Handles side effects by accepting a function that contains imperative, possibly effectful code.
2. useEffect()
(componentDidUpdate):
- An optional second parameter can be passed to specify dependencies, and if any of these dependencies change, the effect is re-run.
- If you want an effect to run after every render (including the initial one), you can omit the dependency array. This simulates the behavior of componentDidUpdate().
3.useEffect()
conditional (componentDidUpdate):
- You can also specify dependencies in the dependency array to control when the effect runs, simulating the behavior of componentDidUpdate() with conditions.
- These depedencies could be variables that can be change over time
4. useEffect()
cleanup(componentWillUnmount):
- To perform cleanup tasks when the component is unmounted, return a function from within the effect function.
- This function will be invoked when the component is unmounted or before re-running the effect.